原题干:
There is a public bike service in Hangzhou City which provides great convenience to the tourists from all over the world. One may rent a bike at any station and return it to any other stations in the city.
The Public Bike Management Center (PBMC) keeps monitoring the real-time capacity of all the stations. A station is said to be in perfectcondition if it is exactly half-full. If a station is full or empty, PBMC will collect or send bikes to adjust the condition of that station to perfect. And more, all the stations on the way will be adjusted as well.
When a problem station is reported, PBMC will always choose the shortest path to reach that station. If there are more than one shortest path, the one that requires the least number of bikes sent from PBMC will be chosen.
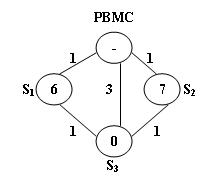
Figure 1
Figure 1 illustrates an example. The stations are represented by vertices and the roads correspond to the edges. The number on an edge is the time taken to reach one end station from another. The number written inside a vertex S is the current number of bikes stored at S. Given that the maximum capacity of each station is 10. To solve the problem at S3, we have 2 different shortest paths:
1. PBMC -> S1 -> S3. In this case, 4 bikes must be sent from PBMC, because we can collect 1 bike from S1 and then take 5 bikes to S3, so that both stations will be in perfect conditions.
2. PBMC -> S2 -> S3. This path requires the same time as path 1, but only 3 bikes sent from PBMC and hence is the one that will be chosen.
Input Specification:
Each input file contains one test case. For each case, the first line contains 4 numbers: Cmax (<= 100), always an even number, is the maximum capacity of each station; N (<= 500), the total number of stations; Sp, the index of the problem station (the stations are numbered from 1 to N, and PBMC is represented by the vertex 0); and M, the number of roads. The second line contains N non-negative numbers Ci (i=1,...N) where each Ci is the current number of bikes at Sirespectively. Then M lines follow, each contains 3 numbers: Si, Sj, and Tij which describe the time Tij taken to move betwen stations Si and Sj. All the numbers in a line are separated by a space.
Output Specification:
For each test case, print your results in one line. First output the number of bikes that PBMC must send. Then after one space, output the path in the format: 0->S1->...->Sp. Finally after another space, output the number of bikes that we must take back to PBMC after the condition of Sp is adjusted to perfect.
Note that if such a path is not unique, output the one that requires minimum number of bikes that we must take back to PBMC. The judge's data guarantee that such a path is unique.
Sample Input:
10 3 3 5 6 7 0 0 1 1 0 2 1 0 3 3 1 3 1 2 3 1
Sample Output:
3 0->2->3 0
题目大意:
每个共享自行车站最大容纳量为Cmax,最完美的状态为自行车个数为Cmax/2,如果一个车站的自行车数量是满的或者是空的,那么PBMC(控制中心)就要去派人调度该车站,使之达到车辆数为Cmax/2的完美状态
但是这个去调度车站车辆数目的人很爱管闲事啊,他遇到一个不完美(没有达到或超过Cmax/2)的车站,就会使之达到完美。如果车多了,他就把车收走,如果车少了,他就从PBMC带车给他。如果路上收的车够用,就先拿路上的车。最终处理完应该处理的车站,就会把剩余的车带回。
求最短路径,如果有多条最短路径,则求需要从PBMC拿走车子最少的一条,如果仍然有多条,则找到需要带回PBMC最少的一条。
这道题必须使用Dijkstra+DFS来做。因为带走最少(MinNeed)和带回最少(MinBack)在路径传递不满足最优子结构,不是简单的相加过程。而且逻辑这么复杂。。。
Dijkstra+DFS的介绍看这里:https://www.mmuaa.com/post-218.html
代码如下:
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstdio>
using namespace std;
const int maxn = 510, inf = 0x7fffffff;
//无向图的邻接矩阵,点权
int G[maxn][maxn], V[maxn], Cmax, Cbest, PointCnt, FuckPoint, RoadCnt;
//存放前驱和真实路径的vector
vector<int> pre[maxn], path;
void Dijkstra(int s){
//Dijkstra算法初始化
bool visited[maxn] = {false};
int MinDist[maxn];
fill(MinDist, MinDist + maxn, inf);
MinDist[s] = 0;
pre[0].push_back(0);
//算法开始
for(int i = 0; i < PointCnt; i++){
int p = -1, min = inf;
for(int j = 0; j <= PointCnt; j++)
if(!visited[j] && MinDist[j] < min) p = j, min = MinDist[j];
if(p == -1) return; //图不连通
visited[p] = true; //标记访问
for(int j = 0; j <= PointCnt; j++){
if(!visited[j] && G[p][j] != inf){
//找到同样好的路径,丢到前驱中
if(MinDist[p] + G[p][j] == MinDist[j])
pre[j].push_back(p);
//找到更好的路径,清空前驱,然后丢到前驱中
else if(MinDist[p] + G[p][j] < MinDist[j]){
MinDist[j] = MinDist[p] + G[p][j];
pre[j].clear();
pre[j].push_back(p);
}
}
}
}
}
//存放发出和回收自行车数量的最小值
int MinSent = inf, MinBack = inf;
//存放临时路径
vector<int> tmpPath;
void DFS(int s){ //从终点遍历到起点
tmpPath.push_back(s);
//递归边界:到达起点
if(s == 0){
int sent = 0, back = 0;
for(int i = tmpPath.size() - 1; i >= 0; i--){
if(V[tmpPath[i]] > Cbest) //多了多了
back += V[tmpPath[i]] - Cbest;
else if(V[tmpPath[i]] == Cbest);//正好正好
else if(V[tmpPath[i]] < Cbest){ //少了少了
back -= (Cbest - V[tmpPath[i]]); //前面搜刮的放到这里
if(back < 0){ //防止车子不够,应该从控制中心拿,加到sent里
sent -= back;
back = 0;
}
}
}
if(sent < MinSent){ //需要从控制中心拿的车子更少
MinSent = sent;
MinBack = back;
path = tmpPath;
}else if(sent == MinSent && back < MinBack){ //需要带回控制中心的更少
MinBack = back;
path = tmpPath;
}
}
//递归式
else{
for(int i = 0; i < pre[s].size(); i++)
DFS(pre[s][i]);
}
tmpPath.pop_back();
}
int main(){
cin >> Cmax >> PointCnt >> FuckPoint >> RoadCnt;
Cbest = Cmax / 2;
//初始化图
fill(G[0], G[0] + maxn*maxn, inf);
//读取点权
V[0] = Cbest;
for(int i = 1; i <= PointCnt; i++)
cin >> V[i];
//读取边权
for(int i = 0; i < RoadCnt; i++){
int l, r;
cin >> l >> r;
cin >> G[l][r];
//无向图
G[r][l] = G[l][r];
}
Dijkstra(0);
DFS(FuckPoint);
//输出结果
cout << MinSent << ' ';
for(int i = path.size()-1; i >= 0; i--){
if(i != path.size()-1) cout << "->";
cout << path[i];
}
cout << ' ' << MinBack << endl;
return 0;
}